Freshers .NET Stop:
This is very easiest way of stuff I have gathered to understand & clear basic concepts of .NET
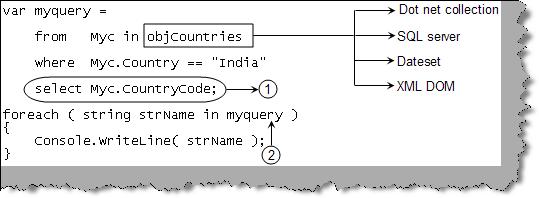
Download Files: from here
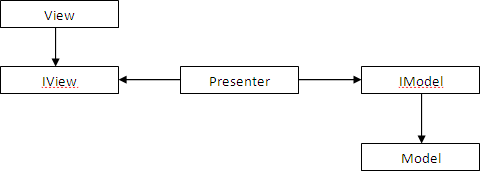

This is very easiest way of stuff I have gathered to understand & clear basic concepts of .NET
.Net framework:
It is a software framework developed by microsoft that runs under Microsoft Windows.It includes large librory and provides language interoperability (i.e each language code can be written in another languages) across several programming languages .Class Library and CLR(common language run time) constitute the .NET framework.
Class libraries are two types in .net that are BCL(base class library) and FCL(framework class library)
The Framework Class Library is everything included in the entire .NET framework installation. This includes ADO.NET, ASP.NET, WPF, Windows Forms, etc.
The Base Class Library is the main core part of the framework, which allows the Common Language Runtime to operate properly. This would include the System namespace, core types, etc.
So the FCL would be considered a superset of the BCL.
CLR
The environment in which managed code executes( managed code runs under CLR and unmanaged code runs under OS). The common language runtime provides just-in-time compilation, enforces type safety, and manages memory through garbage collection.
CLI
Common Language Infrastructure (CLI) is an open specification developed by Microsoft that describes the executable code and runtime environment that form the core of the Microsoft .NET Framework.The specification defines an environment that allows multiple high-level languages to be used on different computer platforms without being rewritten for specific architectures.
CTS
Common type system provides base set of data which is common to all .net languages used by .net framework.
CLS
Common Language specifications provides set of base rules which should be followed all the languages which are supported by .net framework.
The CLS rules define a subset of the Common Type System.
Assembly
Assembly is a logical collection of smallest unit in .Net Framework.
It contains MSIL(microsoft intermediate language which the common language runtime executes) ,manifest that contains the assembly metadata. This metadata contains information about the assembly version, its security identity, the resources required by the assembly, and the scope of the assembly. architectures.
Every Assembly you create contains one or more program files and a Manifest. There are two types program files : Process Assemblies (EXE) and Library Assemblies (DLL)(Your code, compiled into MSIL This code file can be either an EXE file or a DLL file). We can create two types of Assembly, private Assembly and shared Assembly. A private Assembly is used only by a single application, and usually it is stored in that application's install directory. A shared Assembly is one that can be referenced by more than one application. If multiple applications need to access an Assembly, we should add the Assembly to the Global Assembly Cache (GAC)(The global assembly cache stores assemblies specifically designated to be shared by several applications on the computer).
Page Life Cycle((or) methods which are fired during the page load event):(Majorly)
Init() - when the page is instantiated
Load() - when the page is loaded into server memory
PreRender() - the brief moment before the page is displayed to the user as HTML
Unload() - when page finishes loading.
For more in detail of Page Life cycle please refer my post about ASP.NET Page Life Cycle
For more in detail of Page Life cycle please refer my post about ASP.NET Page Life Cycle
Response.Redirect sends message to browser saying that the browser should request some other page so basically it means that:
1. Browser is on page default1.aspx on which there is a Response.Redirect("") command which means that the server sends response (message) to browser that browser should request some other page default2.aspx
2. Browser sends request to server in order to get this page default2.aspx
3. Server sends page default2.aspx to browser this is round trip it means that Response.Redirect has round trip.
Server.Transfer doesn't tell the browser to request page default2.aspx. It just sends page default2.aspx, so e.g., browser address bar still shows the original page's URL.
It means that it doesn't has the round trip.
Roundtrip is the combination of a request being sent to the server and response being sent back to browser.
Response.Redirect vs Server.Transfer
- Response.Redirect involves a roundtrip to the server whereas Server.Transfer conserves server resources by avoiding the roundtrip. It just changes the focus of the webserver to a different page and transfers the page processing to a different page.
- Response.Redirect can be used for both .aspx and html pages whereas Server.Transfer can be used only for .aspx pages.
- Response.Redirect can be used to redirect a user to an external websites. Server.Transfer can be used only on sites running on the same server. You cannot use Server.Transfer to redirect the user to a page running on a different server
- Response.Redirect changes the url in the browser. So they can be bookmarked. Whereas Server.Transfer retains the original url in the browser. It just replaces the contents of the previous page with the new one.
State Management
State management is done at client side and server side
Client Side: Client Side it can achieved with the help of View state, Cookies, Query String,hidden fields and control state.
Server Side: with the help of Cache, Application,Session and Database.
static assembly
A static assembly is created when you compile the program using any of the .NET language compilers.A static assembly is stored on the hard disk in the form of a portable executable (.exe or .dll) file.
private assembly
When you deploy an assembly which can be use by single application, than this assembly is called a private assembly.
Private assemblies can be used by only one application they are deployed with.
Private assemblies are deployed in the directory where the main application is installed.
shared assembly
When you deploy an assembly which can be used by several application, than this assembly is called shared assembly.
Shared assemblies are stored in a special folder called Global Assembly Cache (GAC), which is accessible by all applications.
Access Specifiers or Modifiers:
I would say access specifiers are the one which decides scope of class members.
Let us discuss its different types and scope with beautiful examples:
First see this below I have declared each access specifiers and see its examples step by step
in order to understand it completely.
namespace AccessSpecifiers
{
public class Class1
{
public void display1()
{
Console.WriteLine("public Access Specifier");
}
private void display2()
{
Console.WriteLine("Private Access Specifier");
}
internal void display3()
{
Console.WriteLine("internal Access Specifier");
}
protected void display4()
{
Console.WriteLine("protected Access Specifier");
}
protected internal void display5()
{
Console.WriteLine("protected internal Access Specifier");
}
}
}
private Access Specifier or Modifier:
The scope of the private members is limited within the Class or Structure in which they are defined. They are not accessible outside the Class or Structure.
In the attached example, we have display2() method which is defined as private in Access Specifiers project, Class1.cs class.
We tried to access this method in the Class2.cs class and Class3.cs class (which is derived from Class1.cs class). But it produces the error as AccessSpecifiers.Class1.display2() is inaccessible due to its protection level.
class Class2
{
void display()
{
Class1 obj = new Class1();
obj.display2(); //Error, because display2() is private
}
}
class Class3:Class1
{
void display()
{
display2(); //Error, because display2() is private
}
}
public Access Specifier or Modifier:
Public members have no access restriction. You can access public members by creating object, in derived class and with in the assembly and in other assemblies also.
We have display1() method which is defined as public in AccessSpecifiers project, Class1.cs class. We can access this method in Class2.cs class by creating object, in Class3.cs class which is derived from Class1.cs class and also in other assembly AccessSpecifiersTest also.
namespace AccessSpecifiers
{
class Class2
{
void display()
{
Class1 obj = new Class1();
obj.display1(); //No Error, because display1() public
}
}
}
namespace AccessSpecifiers
{
class Class3:Class1
{
void display()
{
display1(); //No Error, because display1() public
}
}
}
In other assembly AccessSpecifiersTest,
namespace AccessSpecifiersTest
{
class Program
{
static void Main(string[] args)
{
//try to access AccessSpecifier Assembly methods
AccessSpecifiers.Class1 obj = new AccessSpecifiers.Class1();
obj.display1(); //public method, No Error
}
}
}
protected Access Specifier or Modifier:
You cannot access protected members directly i.e., you cannot access protected members by creating object. You can access protected members in derived class only.
We have display4() method which is defined as protected in AccessSpecifiers project, Class1.cs class. We can access this method in Class3.cs class which is derived from Class1.cs class and elsewhere you cannot access this method.
namespace AccessSpecifiers
{
class Class2
{
void display()
{
Class1 obj = new Class1();
obj.display4(); //Error, because display4() is protected
}
}
}
namespace AccessSpecifiers
{
class Class3:Class1
{
void display()
{
display4(); //No Error, because display4() is protected
}
}
}
namespace AccessSpecifiersTest
{
class Program
{
static void Main(string[] args)
{
//try to access AccessSpecifier Assembly methods
AccessSpecifiers.Class1 obj = new AccessSpecifiers.Class1();
obj.display4();//Error, because display4() is protected
}
}
}
internal Access Specifier or Modifier:
Internal members are accessible with in the assembly only. You cannot access internal members outside of the assembly.
We have display3() method which is defined as internal in AccessSpecifiers project, Class1.cs class. We can access this method in Class2.cs class by creating object, in Class3.cs class which is derived from Class1.cs class. Because Class2.cs and Class3.cs classes are in the same assembly AccessSpecifiers. You cannot access display3() method other assembly AccessSpecifiersTest, it produces compile time error.
namespace AccessSpecifiers
{
class Class2
{
void display()
{
Class1 obj = new Class1();
obj.display3(); //No Error, because display3() is internal
}
}
}
namespace AccessSpecifiers
{
class Class3:Class1
{
void display()
{
display3(); //No Error, because display3() is internal
}
}
}
namespace AccessSpecifiersTest
{
class Program
{
static void Main(string[] args)
{
//try to access AccessSpecifier Assembly methods
AccessSpecifiers.Class1 obj = new AccessSpecifiers.Class1();
obj.display3();//Error, because display3() is internal
}
}
}
protected internal Access Specifier or Modifier:
Protected internal scope is addition of protected and internal scope. That means protected internal members are accessible in the derived class as well as with in the assembly.
We have display5() method which is defined as protected internal in AccessSpecifiers project, Class1.cs class. We can access this method in Class2.cs class by creating object, in Class3.cs class which is derived from Class1.cs class, but we cannot access in other assembly AccessSpecifiersTest.
namespace AccessSpecifiers
{
class Class2
{
void display()
{
Class1 obj = new Class1();
obj.display5(); //No Error, because display5() is protected internal
}
}
}
namespace AccessSpecifiers
{
class Class3:Class1
{
void display()
{
display5(); //No Error, because display5() is protected internal
}
}
}
namespace AccessSpecifiersTest
{
class Program
{
static void Main(string[] args)
{
//try to access AccessSpecifier Assembly methods
AccessSpecifiers.Class1 obj = new AccessSpecifiers.Class1();
obj.display5();//Error, because display5() is protected internal
}
}
}
Conclusion:
1.Access specifiers are the one which defines scope.
2.Public has scope everywhere irrespective of assemble and classes
3.Private scope is within the class
4.Protected scope is in the derived class
5.Internal scope is within the same assembly
6.Internal protected scope is internal+protected scope
----------------------------------------------------------------------------------------------------------------------------
Sealed classes and Sealed methods:
If Sealed key word used with class then it means that it won’t be inherited.in the same way if it used with method then it won’t be inherited.But sealed methods overrides an inherited virtual method with the same signature.to understand see below example:
Ex:
class A
{
public virtual void First()
{
Console.WriteLine("First Class A");
}
public virtual void Second()
{
Console.WriteLine("Second Class A");
}
}
class B : A
{
public sealed override void First()
{
Console.WriteLine("First Class B");
}
public override void Second()
{
Console.WriteLine("Second Class B");
}
}
class C : B
{
public override void Second()
{
Console.WriteLine("First Class C");
} //here you cannot inherit void First() as it has Sealed keyword
}
Example: Test1.cs:-
namespace PartialClass
{
public partial class MyTest
{
private int a;
private int b;
public void getAnswer(int a, int b)
{ this.a = a; this.b = b; }
}
}
Test2.cs:-
namespace PartialClass
{
public partial class MyTest
{
public void PrintCoOrds()
{
Console.WriteLine("Integervalues: {0},{1}", a, b);
Console.WriteLine("Addition: {0}", a+b);
Console.WriteLine("Mulitiply: {0}", a * b);
} } }
Program.cs:-
namespace PartialClass
{
class Program
{
static void Main(string[] args)
{
MyTest ts = new MyTest();
ts.getAnswer(12, 25);
ts.PrintCoOrds();
Console.Read();
} } }
OUTPUT:
Integer values: 12,25
Addition: 37
Multiply: 300
Advantages:
- More than one Programmer or developer can simultaneously write the code for class.
- Partial class allows a clean separation of business logic layer and the user interface.
Partial class or struct can contain Partial method.
A partial method declaration consists of two parts:
1. definition and
2. Implementation.
partial void onNameChanged();
// Implementation
partial void onNameChanged() { // method body }
Interface
An interface is like a class but all the methods and properties are abstract. An Interface cannot be instantiated like abstract class. All the methods and properties defined in Interface are by default public and abstract.
Interface generally refers to an abstraction that an entity provides of itself to the outside. Interface can help in separating the methods for external and internal communication without effecting in the way external entities interact with the type..
Abstract Class
An abstract class is a class with at least one method defined as abstract. This type of class cannot be instantiated. An abstract class can have one or more abstract methods and properties and other methods and properties like normal classes.
Now let us understand it and its differences through good examples
"Difference Between abstract Class and Interface" is the important question being asked in .Net world . I will explain the difference theoretically followed by example.
Theoretically there are basically 5 differences between Abstract Class and Interface which are listed as below :-
A class can implement any number of interfaces but a subclass can at most use only one abstract class.
An abstract class can have non-abstract Methods(concrete methods) while in case of Interface all the methods has to be abstract.
An abstract class can declare or use any variables while an interface is not allowed to do so.
So following Code will not compile :-
Interface TestInterface
{
int x = 4; // Filed Declaration in Interface
void getMethod();
string getName();
}
abstract class TestAbstractClass
{
int i = 4;
int k = 3;
public abstract void getClassName();
}
It will generate a compile time error as :-
Error 1 Interfaces cannot contain fields .
So we need to omit Field Declaration in order to compile the code properly.
interface TestInterface
{
void getMethod();
string getName();
}
abstract class TestAbstractClass
{
int i = 4;
int k = 3;
public abstract void getClassName();
}
Above code compiles properly as no field declaration is there in Interface.
An abstract class can have constructor declaration while an interface can not do so.
So following code will not compile :-
interface TestInterface
{
// Constructor Declaration
public TestInterface()
{
}
void getMethod();
string getName();
}
abstract class TestAbstractClass
{
public TestAbstractClass()
{
}
int i = 4;
int k = 3;
public abstract void getClassName();
}
Above code will generate a compile time error as :-
Error 1 Interfaces cannot contain constructors
So we need to omit constructor declaration from interface in order to compile our code .
Following code compile s perfectly :-
interface TestInterface
{
void getMethod();
string getName();
}
abstract class TestAbstractClass
{ public TestAbstractClass()
{
}
int i = 4;
int k = 3;
public abstract void getClassName();
}
An abstract Class is allowed to have all access modifiers for all of its member declaration while in interface we can not declare any access modifier(including public) as all the members of interface are implicitly public.
Note here I am talking about the access specifiers of the member of interface and not about the interface.
Following code will explain it better :-
It is perfectly legal to give provide access specifier as Public (Remember only public is allowed)
public interface TestInterface
{
void getMethod();
string getName();
}
Above code compiles perfectly.
It is not allowed to give any access specifier to the members of the Interface.
interface TestInterface
{
public void getMethod();
public string getName();
}
Above code will generate a compile time error as :-
Error 1 The modifier 'public' is not valid for this item.
But the best way of declaring Interface will be to avoid access specifier on interface as well as members of interface.
interface Test
{
void getMethod();
string getName();
}
InterFace Brief Explanation
An interface contains only the signatures of methods, delegates or events. The implementation of the methods is done in the class that implements the interface, as shown in the following example:
interface ISampleInterface
{
void SampleMethod();
}
class ImplementationClass : ISampleInterface
{
// Explicit interface member implementation:
void ISampleInterface.SampleMethod()
{
// Method implementation.
}
static void Main()
{
// Declare an interface instance.
ISampleInterface obj = new ImplementationClass();
// Call the member.
obj.SampleMethod(); }
}LINQ(Language Integrated Query):
I want you to understand LINQ in a simple and best way.
LINQ is a uniform programming model for any kind of data access.
LINQ enables you to query and manipulate data independently of data sources.
(Data Source could be any it allows common query to fetch the data)
Below figure 'LINQ' shows how .NET language stands over LINQ programming model and works in a uniformed manner over any kind of data source. I
It’s like a query language which can query any data source and any transform. LINQ also provides full type safety and compile time checking.
LINQ can serve as a good entity for middle tier. So it will sit in between the UI and data access layer.
Below figure Explains you that Linq is giving you the facility to access data from different data sources with a common query.
Below is a simple sample of LINQ. We have a collection of data ‘objcountries’ to which LINQ will is making a query with country name ‘India’. The collection ‘objcountries’ can be any data source dataset, datareader, XML etc. Below figure ‘LINQ code snippet’ shows how the ‘ObjCountries’ can be any can of data. We then query for the ‘CountryCode’ and loop through the same.
Even If you have not understand LINQ let us see below where I am explaning LINQ by comparing general query as always write.
I have table1 which has columnd id ,cityname,countryname
I want all the cities for india,So,I will write it as follows
GeneralWay:Select Cities From table1 where countryname=’india’
now,with LINQ it can be
var anyobj = from temptable in table1 where countryname==”india”
Select temptable.Cities
first way allows you to fetch data from SQL only.
But LINQ query is common to all databases may be SQL,Oracle,Excel..etv
I am gonna give you the link thats below, which is v.Excellent in order to know about the linq opertaions in vb.net and c#.net with good examples:
For more example see this links:
You can see the videos of it part by part on youtube by typing
LINQ on Youtube Uploaded by cam2149 or here are the links
It has very good Explanation
Entity framework ORM(Object Relational Mapping):
Entity Framework (EF) is what is known as an Object Relational Mapper (ORM).
ORM is simply the concept of processing data from a relational database and modeling it in a format that is more readily usable by your code. Instead of looping through rows in a table you model each row as an object and loop through a collection of objects, called entities.
In .NET there are two different ORM products that do basically the same thing. The first one is LINQ to SQL (L2S). Second we have Entity Framework. L2S is the older of the two technologies. At their core they both accomplish the same goal, but L2S has quite a few more limitations than EF. The most obvious limitation is that L2S only works with a SQL Server database.
The ultimate goal of ORMs in .NET is to remove the need to execute string queries and give the developer the ability to write strongly-typed queries. If you're familiar with LINQ then you already know how to write strongly-typed queries against IEnumerable<T> collections. The benefit of writing a strongly-typed query is that the compiler can look at a LINQ query and tell you if you made a mistake. Of course now and then you'll still run into runtime errors, but the benefit of compiled queries is immense.
The ORM's job is to take your LINQ statements and translate them into SQL queries for you behind the scenes. This enables a developer to write complex queries against the database without ever having to write a single line of SQL. This does have the potential to allow SQL ignorant developers to remain ignorant but I think that is a small risk compared to the advantages it provides.
The simplest way to explain ORM is to first see how we would access data without it.
The Old Way(without entities)I will show you how to access a database in C# the old fashioned way, using plain old ADO.NET. Here are the steps you would need in order to connect to a SQL server database:
First you need a SqlConnection object.
SqlConnection connection = new SqlConnection(connectionString);
If you've never seen a connection string before, it's just a string containing all the authentication data necessary to connect to the desired database. Here is a sample of what a connection string looks like.
Once you have a connection, you need a SqlCommand object.
SqlCommand command = new SqlCommand(@"INSERT INTO [CodeTunnel].[Users]
VALUES (@Username, @Password, @Administrator)", connection);
VALUES (@Username, @Password, @Administrator)", connection);
Now we have to pass in the parameters by adding them to the Parameters collection on the SqlCommand.
command.Parameters.Add(new SqlParameter("Username", "Alex"));
command.Parameters.Add(new SqlParameter("Password", "Exp3rtC0d3r1"));
command.Parameters.Add(new SqlParameter("Administrator", true);
command.Parameters.Add(new SqlParameter("Password", "Exp3rtC0d3r1"));
command.Parameters.Add(new SqlParameter("Administrator", true);
Then you have to open the connection to the underlying database.
connection.Open();
Execute the command.
int rowsAdded = command.ExecuteNonQuery();
Console.WriteLine("{0} rows were added.", rowsAdded);
Console.WriteLine("{0} rows were added.", rowsAdded);
Finally you have to close the connection to the database.
connection.Close();
The New Way(through entities)
Instantiate the data context object.
CodeTunnelEntities dataContext = new CodeTunnelEntities();
// EF will automatically read the connection string from your application configuration file :)
// EF will automatically read the connection string from your application configuration file :)
Create a new User object.
User newUser = new User
{
Username = "Alex",
Password = "Exp3rtC0d3r1",
Administrator = true
};
{
Username = "Alex",
Password = "Exp3rtC0d3r1",
Administrator = true
};
Add the user to the data context.
dataContext.Users.AddObject(newUser);
Save changes to the data context in order to persist those changes to the database.
dataContext.SaveChanges();
References:(recommended to get more details easily)
Good example to start with entity framework at first time(v).
To learn completely entity frame work keep on watching the video of different parts in sequence.I have listed 3 videos in sequence from start:(v)
http://www.youtube.com/watch?v=BS6IKdUd2V8&feature=relmfu
Theory link to start with orm or entities
http://www.codetunnel.com/blog/post/73/introduction-to-entity-framework-part-i--object-relational-mapping
http://www.codetunnel.com/blog/post/75/introduction-to-entity-framework-part-iii--building-the-model
Structure & Classes:
I would give you the simple example to illustrate about the structure & classes,structure is nothing but user defined data type,considered as value type data types,uses stack memory.Stack memory is used to store values.
Now let us come to class,it is almost use for the above purpose but it is considered as reference type data type uses Heap memory,heap memory will store the reference or memory address.
struct exstuct
{
public int a;
public void Display()
{
Console. WriteLine(this.a);
}
}
Static void main()
{
exstuct val;
val.a =10;
val.Display();
Console.Read();
}
o/p:10
MVP excellent Explanation with good example
These days design patterns such as MVC and MVP in ASP.Net are being used in Enterprises. When I heard of these terms I searched Google and read books but I was always confused because the examples given were quite complex. Then recently I worked on a project of MVP and then I understood all the insides in it and decided to write a small and simple article for understanding MVP. So here we'll discuss the MVP pattern. The MVP is Model, View , Presenter. This pattern is how the interaction between these layers can be done.
View: View can be your Aspx page in your web applications or any user controls/Interface for the end user.
Model: Contains all the business logic.
Presenter: Works as the intermediate agent for Model and View. It binds the view with the model. See the diagram below. 
In the following diagram a couple of blocks are added which are interfaces through which the presenter will interact.
Let's take a simple example of how to implement it.
Create a web application project in your Visual Studio. Now add 3 more classes named View.cs, Presenter.cs and Model.cs and add the interfaces IView and IModel.
Start with the aspx page (View). Add a label, a button and a TextBox.
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="Label1" runat="server" Text="Label"></asp:Label>
<asp:TextBox ID="TextBox1" runat="server"></asp:TextBox>
</div>
<asp:Button ID="Button1" runat="server" Text="Button" onclick="Button1_Click" />
</form>
</body>
</html>
Now we won't write anything in aspx.cs file. First write the code in IView.
namespace WebApplication1
{
public interface IView
{
String Label { get; set; }
String TextBox { get; set; }
}
}
Similarly write some code in IModel.cs
namespace WebApplication1
{
public interface IModel
{
List<String> setInfo();
}
}
Now Model.cs. Let's say we send the information about the Label and TextBox from Model.
namespace WebApplication1
{
class Model : IModel
{
public List<String> setInfo()
{
List<String> l = new List<string>();
l.Add("Enter Name:");
l.Add("Use capital letter only");
return l;
}
}
}
Now we need to write some code in the presenter so that the View and Model can communicate with each other. It'll go like this:
namespace WebApplication1
{
public class Presenter
{
IView _pView;
IModel _pModel;
public Presenter(IView PView, IModel PModel)
{
_pView = PView;
_pModel = PModel;\
}
public void BindModalView()
{
List<String> ls = _pModel.setInfo();
_pView.Label = ls[0];
_pView.TextBox = ls[1];
}
}
}
Finally go to aspx.cs and Implement the IView.
public partial class _Default : System.Web.UI.Page, IView
{
protected void Page_Load(object sender, EventArgs e)
{
}
#region IView Members
public string Label
{
get
{
return Label1.Text;
}
set
{
Label1.Text = value;
}
}
public string TextBox
{
get
{
return TextBox1.Text;
}
set
{
TextBox1.Text = value;
}
}
#endregion
Add an event for the button and write the code to get the data from Model through presenter and then bind it to the View. In the constructor of Presenter we passed "this" which means reference of the same aspx page.
protected void Button1_Click(object sender, EventArgs e)
{
Presenter p = new Presenter(this, new WebApplication1.Model());
p.BindModalView();
}
And you have implemented a small application with MVP pattern. Now I want to add a few more lines to show the advantage of adding IView and IModel interface. Using these interface your code will be more manageable and reusable. Moreover if you want to test your application you can create a TestProject and can use the IView and IModel for Mock Implementation. And unit test your application.
In the next part of this article I'll try to show you how to test this application using NUnit or any other tool like MockUp for Unit testing.
Hope this was helpful.
Web Service vs WCF and What are they...In Detail
Webservice is a application logic accessible via standard web protocols,One of the standard web protocol is the SOAP.
SOAP is the W3C submitted note that uses XML for data sharing and HTTP for transport.
* It means normal web service must share data in XML with HTTP protocol.
If I would like to explain you in very simple way then I can say ,simple and basic difference is that ASMX web service is designed to send and receive messages using SOAP over HTTP only. While WCF service can exchange messages using any format (SOAP is default) over any transport protocol (HTTP, TCP/IP, MSMQ, NamedPipes etc).
ASMX is simple but limited in many ways as compared to WCF.
- ASMX web services can be hosted only in IIS while WCF service has all the following hosting options:
- a. IIS
- b. WAS (Windows Process Activation Services)
- c. Console Application
- d. Windows NT Services
- e. WCF provided Host
- ASMX web services support is limited to HTTP while WCF supports HTTP, TCP, MSMQ, NamedPipes.
- ASMX Security is limited. Normally authentication and authorization is done using IIS and ASP.NET security configuration and transport layer security.For message layer security, WSE can be used.
- WCF provides a consistent security programming model for any protocol and it supports many of the same capabilities as IIS and WS-* security protocols, additionally, it provides support for claim-based authorization that provides finer-grained control over resources than role-based security.WCF security is consistent regardless of the host that is used to implement WCF service.
- Another major difference is that ASMX web services uses XmlSerializer for serialization while WCF uses DataContractSerializer which is far better in performance than XmlSerializer.
- Key Issues with XmlSerializer in serializing .NET types to xml are:
- a. Only public fields or properties of the .NET types can be translated to Xml.
- b. Only the classes that implement IEnumerable can be translated.
- c. Classes that implement IDictionary, such as Hashtable cannot be serialized.
Further more in details if you want to know,then see below:
Difference between WCF and Web service:
Web service is a part of WCF. WCF offers much more flexibility and portability to develop a service when comparing to web service. Still we are having more advantages over Web service, following table provides detailed difference between them.
Features
|
Web Service
|
WCF
|
Hosting
|
It can be hosted in IIS
|
It can be hosted in IIS, windows activation service, Self-hosting, Windows service
|
Programming
|
[WebService] attribute has to be added to the class
|
[ServiceContract] attribute has to be added to the class
|
Model
|
[WebMethod] attribute represents the method exposed to client
|
[OperationContract] attribute represents the method exposed to client
|
Operation
|
One-way, Request- Response are the different operations supported in web service
|
One-Way, Request-Response, Duplex are different type of operations supported in WCF
|
XML
|
System.Xml.serialization name space is used for serialization
|
System.Runtime.Serialization namespace is used for serialization
|
Encoding
|
XML 1.0, MTOM(Message Transmission Optimization Mechanism), DIME, Custom
|
XML 1.0, MTOM, Binary, Custom
|
Transports
|
Can be accessed through HTTP, TCP, Custom
|
Can be accessed through HTTP, TCP, Named pipes, MSMQ,P2P, Custom
|
Protocols
|
Security
|
Security, Reliable messaging, Transactions
|
WCF(Windows Communication Foundation)You might have got the idea about wcf by reading above,now to start with WCF let us we’ll clarify or discuss some basic and very important concepts about WCF.
endpoints:
WCF service always exposes endpoints its like a portal for communicating with the world,WCF communication always takes place through endpoints.
* endpoints consist of three components
--address == where to communicate
--binding == how to communicate
--contract == with whom to communicate
see the actual example which demonstrates how does endpoints are used in real time:
<system.serviceModel>
<services>
<service name="MathService"
behaviorConfiguration="MathServiceBehavior">
<endpoint
address="http://localhost:8090/MyService/MathService.svc" contract="IMathService"
binding="wsHttpBinding"/>
</service>
</services>
<behaviors>
<serviceBehaviors>
<behavior name="MathServiceBehavior">
<serviceMetadata httpGetEnabled="True"/>
<serviceDebug includeExceptionDetailInFaults="true" />
</behavior>
</serviceBehaviors>
</behaviors>
</system.serviceModel>
<services>
<service name="MathService"
behaviorConfiguration="MathServiceBehavior">
<endpoint
address="http://localhost:8090/MyService/MathService.svc" contract="IMathService"
binding="wsHttpBinding"/>
</service>
</services>
<behaviors>
<serviceBehaviors>
<behavior name="MathServiceBehavior">
<serviceMetadata httpGetEnabled="True"/>
<serviceDebug includeExceptionDetailInFaults="true" />
</behavior>
</serviceBehaviors>
</behaviors>
</system.serviceModel>
fig: demonstrates end point and wcf architecture
After EndPoint I will explain you very in short about Contracts:
Service Contract:
Service contract describes the operation that service provide. Service Contract can be define using [ServiceContract] and [OperationContract] attribute.
ex:
[ServiceContract()]
public interface ISimpleCalculator
{
[OperationContract()]
int Add(int num1, int num2);
}
public interface ISimpleCalculator
{
[OperationContract()]
int Add(int num1, int num2);
}
Once we define Service contract in the interface, we can create implement class for this interface.
public class SimpleCalculator : ISimpleCalculator
{
public int Add(int num1, int num2)
{
return num1 + num2;
}
}
{
public int Add(int num1, int num2)
{
return num1 + num2;
}
}
Data Contract: Its to define your own object or data types rather than int,string..etc,see the example below you will understand.
[ServiceContract]
public interface IEmployeeService
{
[OperationContract]
Employee GetEmployeeDetails(int EmpId);
}
[DataContract]
public class Employee
{
private string m_Name;
private int m_Age;
[DataMember]
public string Name
{
get { return m_Name; }
set { m_Name = value; }
}
[DataMember]
public int Age
{
get { return m_Age; }
set { m_Age = value; }
}
}
public interface IEmployeeService
{
[OperationContract]
Employee GetEmployeeDetails(int EmpId);
}
[DataContract]
public class Employee
{
private string m_Name;
private int m_Age;
[DataMember]
public string Name
{
get { return m_Name; }
set { m_Name = value; }
}
[DataMember]
public int Age
{
get { return m_Age; }
set { m_Age = value; }
}
}
Message Contract
Default SOAP message format is provided by the WCF runtime for communication between Client and service. If it is not meeting your requirements then we can create our own message format. This can be achieved by using Message Contract attribute.
ex:
Message contract can be applied to type using MessageContract attribute. Custom Header and Body can be included to message using 'MessageHeader' and 'MessageBodyMember'atttribute. Let us see the sample message contract definition.
[MessageContract]
public class EmployeeDetails
{
[MessageHeader]
public string EmpID;
[MessageBodyMember]
public string Name;
[MessageBodyMember]
public string Designation;
[MessageBodyMember]
public int Salary;
[MessageBodyMember]
public string Location;
}
public class EmployeeDetails
{
[MessageHeader]
public string EmpID;
[MessageBodyMember]
public string Name;
[MessageBodyMember]
public string Designation;
[MessageBodyMember]
public int Salary;
[MessageBodyMember]
public string Location;
}
When I use this EmployeeDeatils type in the service operation as parameter. WCF will add extra header call 'EmpID' to the SOAP envelope. It also add Name, Designation, Salary, Location as extra member to the SOAP Body.
Fault Contract
Suppose the service I consumed is not working in the client application. I want to know the real cause of the problem. How I can know the error? For this we are having Fault Contract. Fault Contract provides documented view for error occurred in the service to client. This helps us to easy identity, what error has occurred.
ex:
Step 1:
//Service interface
[ServiceContract()]
public interface ISimpleCalculator
{
[OperationContract()]
int Add(int num1, int num2);
}
//Service implementation
public class SimpleCalculator : ISimpleCalculator
{
public int Add(int num1, int num2)
{
//Do something
throw new Exception("Error while adding number");
}
}
[ServiceContract()]
public interface ISimpleCalculator
{
[OperationContract()]
int Add(int num1, int num2);
}
//Service implementation
public class SimpleCalculator : ISimpleCalculator
{
public int Add(int num1, int num2)
{
//Do something
throw new Exception("Error while adding number");
}
}
Step 2: Now if you want to send exception information form service to client, you have to use FaultException as shown below.
public int Add(int num1, int num2)
{
//Do something
throw new FaultException("Error while adding number");
}
{
//Do something
throw new FaultException("Error while adding number");
}
Step 3: Output window on the client side is show below.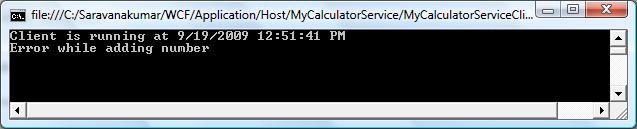
No comments:
Post a Comment